Hey All,
I wrote a little service last night as Reef Pi was unable to control my co2 Scrubber with Reef Pi.
This was just thrown together and I as I normally write in Java and I will continue working on this when I get the time.
I use a a motorised ball valve you can find here Motorized Ball Valve
Basically this unit only has 3 wires so it requires a relay to work.
I used a 240v one with a 240v Relay. So power on will make the valve open power off would make it close.
I also Used Pushover for push notifications.
This valve is attached to a 3 way T.
T is as follows
1: To Skimmer air intake
2: Co2 Scrubber
3: Ball Valve
When valve it closed air will be drawn through the scrubber
When valve is open air will take the path of least resistance through the valve (Saves a hell of alot of media)
Code is below for whoever wants to use it.
Also Check if Media is expired and will send a push notification to your phone
Also check if the skimmer cup is full (Currently using an ATO you cannot alert on it being off, or i have missed this along the way)
I wrote a little service last night as Reef Pi was unable to control my co2 Scrubber with Reef Pi.
This was just thrown together and I as I normally write in Java and I will continue working on this when I get the time.
I use a a motorised ball valve you can find here Motorized Ball Valve
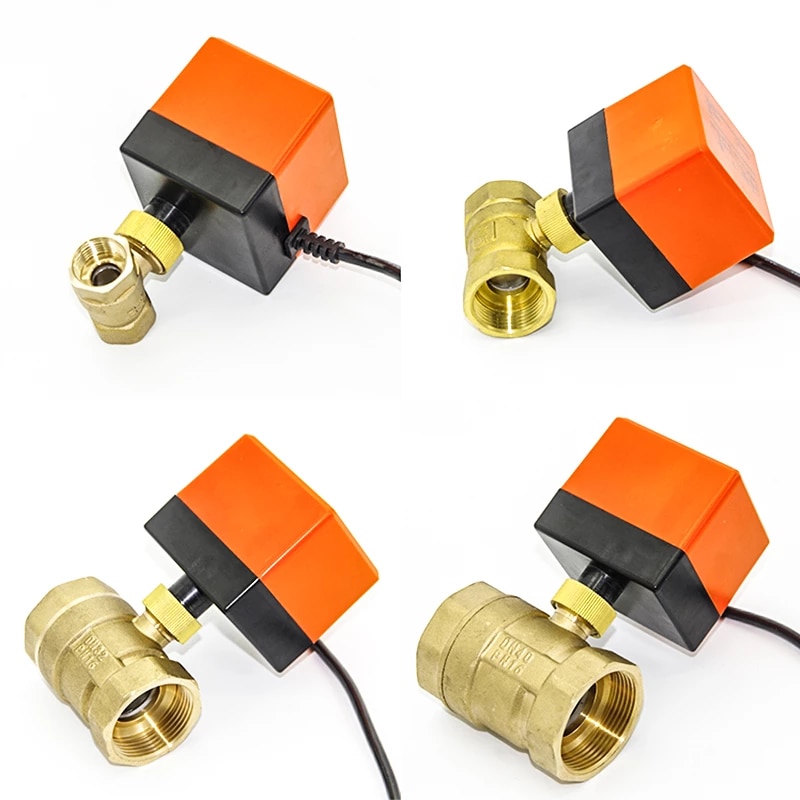
Basically this unit only has 3 wires so it requires a relay to work.
I used a 240v one with a 240v Relay. So power on will make the valve open power off would make it close.
I also Used Pushover for push notifications.
This valve is attached to a 3 way T.
T is as follows
1: To Skimmer air intake
2: Co2 Scrubber
3: Ball Valve
When valve it closed air will be drawn through the scrubber
When valve is open air will take the path of least resistance through the valve (Saves a hell of alot of media)
Code is below for whoever wants to use it.
Also Check if Media is expired and will send a push notification to your phone
Also check if the skimmer cup is full (Currently using an ATO you cannot alert on it being off, or i have missed this along the way)